Magic functions are a methodology for creating clean code. They exist in most programming languages such as Kotlin, C++, Python & C#. Magic functions do not exist in, for example, Java, Go & Javascript.
Here are some examples of Magic functions:
class GameTile:
def __init__(self, x, y, plane, clip_type):
self.X = x
self.Y = y
self.Plane = plane
self.ClipType = clip_type
# Overload equality operator (==)
def __eq__(self, other):
return (
self.X == other.X
and self.Y == other.Y
and self.Plane == other.Plane
and self.ClipType == other.ClipType
)
# Overload addition operator (+)
def __add__(self, other):
return GameTile(
self.X + other.X, self.Y + other.Y, self.Plane + other.Plane, self.ClipType
)
def __str__(self):
return f"X: {self.X}, Y: {self.Y}, Plane: {self.Plane}, ClipType: {self.ClipType}"
Here we have a generic GameTile class for a video game. This is a typical implementation, often extending from a coordinate class, though we don’t show that here.
See those double underscores? In Python specifically, these are known as dunder(double underline) methods. But, in all programming languages where these are implemented we simply call them magic functions.
Why? Because they define how our custom objects behave using operators and class conversions.
Here are two Game Tile magical use cases:
if __name__ == "__main__":
tile1 = GameTile(10, 20, 0, "Grass")
tile2 = GameTile(5, 10, 1, "Water")
print(str(tile1 + tile2))
Here we create two game tiles, add their coordinates together, then turn them into a string. When we add the two tiles we are converting tile 1 and tile 2 into another instance of the tile class.
As this may illustrate, another name for magic programming is “operator overloading” or also meta-programming.
This means programming the programming itself.
Take a look at the exact same example in C++:
class GameTile {
public:
int X;
int Y;
int Plane;
std::string ClipType;
GameTile(int x, int y, int plane, const std::string& clipType)
: X(x), Y(y), Plane(plane), ClipType(clipType) {}
// Overload equality operator (==)
bool operator==(const GameTile& other) const {
return (X == other.X) && (Y == other.Y) && (Plane == other.Plane) && (ClipType == other.ClipType);
}
// Overload addition operator (+)
GameTile operator+(const GameTile& other) const {
return GameTile(X + other.X, Y + other.Y, Plane + other.Plane, ClipType);
}
// Overload stream insertion operator (<<) for string conversion
friend std::ostream& operator<<(std::ostream& os, const GameTile& tile) {
os << "X: " << tile.X << ", Y: " << tile.Y << ", Plane: " << tile.Plane << ", ClipType: " << tile.ClipType;
return os;
}
};
It is the exact same implementation but more verbose as C++ usually becomes.
Lets take a look at the results of this meta-programming:
int main() {
GameTile tile1(10, 20, 0, "Grass");
GameTile tile2(5, 10, 1, "Water");
std::cout << tile1 + tile2 << std::endl;
return 0;
}
Pretty interesting right?
This is hyper important. The majority of frameworks in languages that support magic use these types of implementation.
PyTorch, Pandas & Flask in Python, for example, use magic programming to simplify the logical part of programming.
OpenGL extensions, web frameworks, math libraries, etc. in C++ also use these as well.
When I don’t understand something for a framework I peer into its source code to truly understand the logic. Inside I often see these operator/magic implementations.
8 times out of 10 there are magic functions, especially in larger frameworks.
These are essential for creating clean code when creating a framework for other people to use.
How Would You Learn Magical Programming?
Well, you don’t. There is no reason to memorize every magic function for every language. Instead I would just make sure to know the keywords or syntax then figure it out as you read or write.
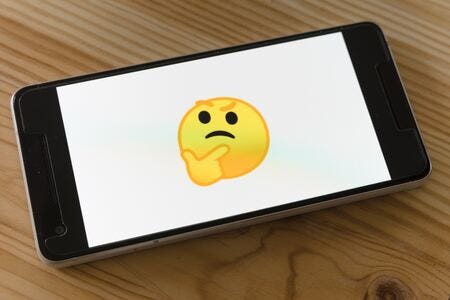
For example, in C++, Kotlin and C# the keyword used is “operator” and in Python the syntax is “dunders” or __str__.
Magic programming is simple and powerful.
It is so simple, there was not much more to write about them. If you understand them from this article, that is all about what you need to know to get programming in them.
Anywho, I hope you learned something…
Happy coding!
Resources
An explanation of abstraction: https://jessenerio.com/how-the-greatest-power-of-code-is-abstraction/