Mastery is a bit of a misnomer. Truly mastering a language, niche or framework means you can find the perfect, most clean and performant, code in all situations. However, there are so many ways to write a piece of code or logic that this typical definition does not apply.
The true definition, the true tipping point, if you will, would be that key moment when you become an “autonomous programmer”.
A coder who can solve nearly any problem with just their notes, AI helper and the internet at their disposal.
This is a good and worthy goal to strive for, “I want to become a coder that can solve 90% of their problems”.
And, by problems I mean a project sliced and diced into 1000s of problems.
If you want to make a website in Java, you split it into a 1000 pieces.
The question is, can you solve 900/1000 of the problems each within a short time?
Even further, did you know, you have to start all over again when you enter a new niche?
Just because you can solve 90% of problems in one niche does not mean you can do the same for another niche.
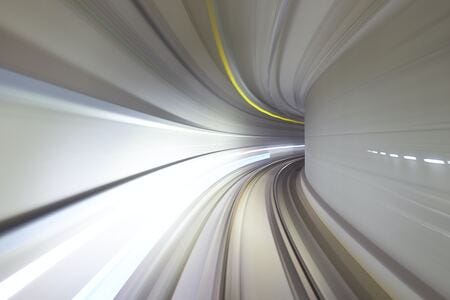
Now, there is transferability. Many of your abilities will transfer over.
Guess… guess what the most transferrable parts of learning a niche is?
It is first the language itself then right after, all the concepts that exist in that niche.
When you transfer niches within the same language, the language knowledge remains and the niche knowledge resets.
When you transfer between languages and stick to the same niche, the concepts of the niche remain the same and the language resets.
The focus of this path here, is, in learning a language.
Java is powerful for creating web apps, APIs, game servers, mobile apps and game development.
Before even getting into these niches or any niche, you should learn the language you are entering.
You will save massive time and effort mastering the language beforehand, especially if it is your first language.
Table of Contents
Start With the Fundamentals
If this is your first time learning to program, the good old fundamentals of Computer Science are your entry point.
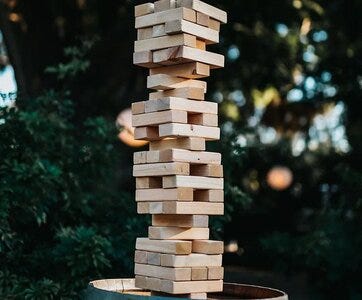
I wrote an in-depth article on why programming fundamentals save time and effort, if you want a deeper read.
But, the goal of focusing on Java here is to maximize transferrable skills. Always, especially at the beginning, you want to focus on the most transferrable skills.
Which are they? It is Computer Science fundamentals then, it is your first language.
What language? Any complete language.
You should have something you are excited about, or at least something motivating about the language you are picking.
It can be machine learning, web development, game development or a specific framework.
Let that guide you.
This article assumes you know you want to learn Java and builds off that.
If you are unsure which language you want to get into, you can get some advice on picking a first language in a previous post.
Now that we got that setup. Here is a good Computer Science fundamentals course I always recommend.
It is evergreen and applies to all programming languages. It is a fantastic start to understanding code.
Pause, take notes, I recommend Codex.
Use an AI helper(any large language model) to verify and explain concepts.
Focus on terminology.
Go Through a Full Course and Do Exercises
I have searched far and wide for the best Java full courses. I spent a good 2 days going through them, returning a few Udemy courses so you don’t have to.
From my research I thought the Udemy courses below were the best for me. But, the YouTube full course was great too.
I have seen people absolutely love and digest full courses I absolutely hated. It is a matter of preference and natural tendencies which you prefer.
This paid Udemy course isn’t necessarily better. It is just a different style in case the YouTube tutorial doesn’t suite you.
For me though, I enjoyed the Udemy course more.
So, next up on your Java conquest. Use either of these two courses:
Free YouTube Full Course
Paid Udemy
https://www.udemy.com/course/java-the-complete-java-developer-course/
Or
https://www.udemy.com/share/1044Co3@0vCDahuc1uYNF4qrf7ubKGNTPxKsUMesOa_hH4DSM70F5GSvFXKjvPTyL9PjhzAc/ (Has homework)
Never pay more than 20% the price.
Often, Udemy has time periods where the courses are less than 20% of the price. It will look like this:

In addition you can look for coupons to get under 20%.
Java is a bit special
Now, I noticed there was an issue with all these courses on Java. Almost all the best ones don’t include enough intermediary exercises for learning Java.
In between concepts or sections there should be some exercises or homework to apply your knowledge.
This is very minimal with all the Java courses I found. If you find a better one than these, email me and let me know.
Without those additional homeworks you may find yourself missing a feeling of support and understanding. I recommend drudging through as much of either course as you can stomach.
Write down the concepts and follow along the code as the course authors write it in the tutorial.
You are going to have some concepts you won’t understand or seem too hard. Just note them down and move forward.
You can refer to smaller individual tutorials to clarify a topic in the full course. Try to understand all of it the best you can. Just make sure to stick to the full course as the main string of lessons.
To help you with the missing homework problem Java has, post a GitHub repository with all the Java course code you are using. Keep notes in a note-taking app. Use an LLM to clarify any concepts you may struggle with.

To get the most out of a full course, do this:
- Take notes (how to take notes in depth)
- Use LLMs (how to use LLMs for learning to code)
- Write along with the course author and post on GitHub
- Follow and finish a full course (Why full courses are so awesome)
- Use smaller tutorials to clarify concepts. Stick to the full course though.
Do exercises
Here is a list of exercises:
- https://www.w3resource.com/java-exercises/ (free)
- https://codegym.cc/prices (expensive)
Every day try to finish 2–5 of them minimum. More is better. For the free version, post them into one solution repository on GitHub if you want. This way you have something to look at in a year.
You will be surprised how simple they look a year later.
All the exercises are important. All of it is used in general programming.
Read the Head First Series and Finish the Java Exercises
I am aware a majority of people won’t stick to this guide up to this point. I have seen how people watch the end of a tutorial series less and less as it progresses.
But, let’s say you got this far.
You will not have a full understanding of Java yet. You will be barely exiting the basics at this point.
My goal is to help you get to the intermediate.
What makes the difference between a beginner and an intermediate is the missing knowledge.
A beginner is like a cheese champion.
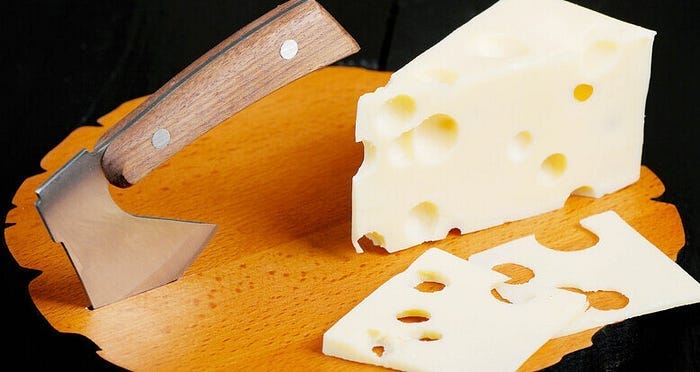
There will be many holes in your knowledge. Headfirst can help you fill those holes really really well.
Here is the book: https://a.co/d/0LOzMda
I will tell you, there are many ways to get books. This book is worth it.
I recommend reading it online and at the same time finish your Java exercises.
If you finish the Java exercises before finishing Head First, then head to the learn Maven section and read while learning Maven
Learn Maven
Maven is the #1 build tool for Java. Every 3rd party library depends on Maven.
It manages dependencies and automates downloading/including them in your project.
Gradle and most other build tools build on top of Maven.
So by learning Maven as your first build tool for Java, you also learn the core lessons needed to understand Gradle, Jenkins & Ant.
You will find the future projects you join will use these build tools and you will be prepared to tackle them because you know the lower level automation tool these are built on, Maven.
Here is a paid and free full course to learn Maven:
There may be some other Udemy courses that more fit your style. Just buy them, go through a few videos, return and buy another if it doesn’t fit.
End With a Niche
Now is the time to finally focus on your niche. In my years coding in Java I have seen some frameworks stick out in the language for each niche. Let me list them out for you:
- Game development: JMonkey Engine (3D), LibGDX (2D)
- Web development: Java Spring
- Mobile development: Android SDK
- GUI Programming: Swing, JavaFX
- Graphics programming: OpenGL
- Specific frameworks: Any other you have in mind
- Etc.
Pick something and finally begin to code what you want. But, at some point it will become a good idea to learn a second language and a new niche. You can start a collection…
Anywho, I hope you learned something…
CTA: Check out my other articles and follow me on medium.
Happy coding!
Resources
Note-taking app: https://codexnotes.com/
Why the fundamentals of coding: https://jessenerio.com/why-the-fundamentals-of-coding-are-the-key-to-success/
How to pick a first language: https://jessenerio.com/how-should-i-pick-a-language-to-start-coding-like-a-superstar/
Introduction to Computer Science: https://youtu.be/zOjov-2OZ0E?si=XgyYNjWTZLt4D-aG
Java Full Free Course: https://youtu.be/xk4_1vDrzzo?si=QadpIJ6KdvugObCJ
Java Udemy Course 1: https://www.udemy.com/course/java-the-complete-java-developer-course/
Java Udemy Course 2: https://www.udemy.com/course/the-complete-java-development-bootcamp/
How to take notes: https://jessenerio.com/how-to-write-a-great-coding-reference-manual-to-teach-your-future-self/
Why use AI for coding: https://jessenerio.com/why-ai-is-one-of-the-best-tools-for-quickly-learning-to-code/
Why use Full Courses: https://jessenerio.com/how-to-study-full-coding-courses/
Java Exercises: https://www.w3resource.com/java-exercises/
Expensive Java Exercises: https://codegym.cc/prices
Head First Java book: https://a.co/d/9x4A8nB
Learn Maven free: https://youtu.be/p0LPfK_oNCM?si=2VgATH7XC_P5lOxw
Learn Maven Udemy: https://www.udemy.com/course/mavencrashcourse/