I want to give you a detailed overview of data structures and algorithms. They are explained to death. But we need them…
Table of Contents
What is a data structure?
A data structure is a set of 0s and 1s. It is memory. But the difference between any piece of memory and a data structure is the organization. Data structures are memory with organized bits.
When we store an integer as x:
int x = 5;
We are reserving a set of bits. It is memorized with structure. The structure in programming is the class integer.
Integer comes with abilities which we can use to leverage code. Let’s create a structure of bits which are organized to have three functions.
For example:
public class MegaInt {
private int value;
public MegaInt(int value) {
this.value = value;
}
public void increment() {
value++;
}
public void decrement() {
value--;
}
public boolean isEven() {
return value % 2 == 0;
}
}
Three functions-increment, decrement and isEven are stored in memory as part of the data structure, MegaInt. We have organized our bits to fit a specific design.
All data structures follow some design like this. Classes, ints, floats, bytes, strings even arrays are all data structures. They each have their own abilites and structure in memory as data.
What is an algorithm?
The key feature of an algorithm is its reproducibility. If we have a problem we solve it with a logical set of steps. For example, lets say we want to reproduce a straight line at 45 degrees:
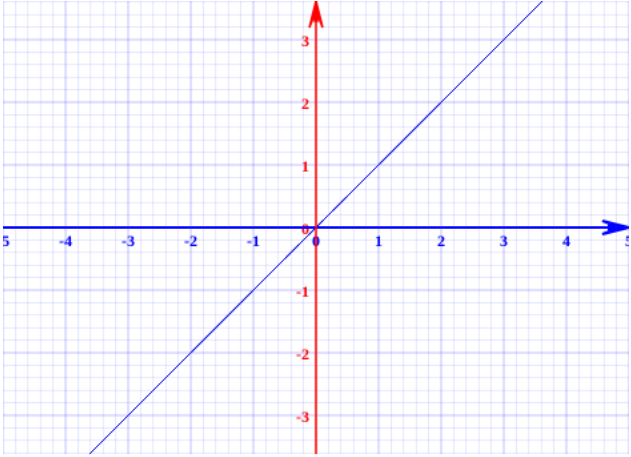
Our algorithm for reproducing this production is f(x) = x. From here on we can say the algorithm for this line is a formula or equation.
In the same way we solve for the line-we solve for real technical challenges using code. The code itself is the formula just like f(x) = x. When we code we are to envision the problem then reverse code to solve it in our mind.
An algorithm is the solution to the problem. Every time you run it-it solves the same problem again and again.
Sounds hard? Well it is. Coding is hard. It takes years to get decent and much longer to get good. But it is the coolest experience to solve problems from your imagination-to create algorithms.
Its all 0s and 1s
For higher level languages we use logic. Programming is so abstracted from the hard 0s & 1s it has come to resemble logic. However in the end all of programming is binary numbers. The off and on of circuitry.
An algorithm takes memory AKA data structures and manipulates them using these numbers. You cannot have an algorithm without memory or memory without an algorithm. They are intertwined as natural law.
How do they work together?
Electricity flows through your entire computer with a rhythm. It is a cycle of electric current going through the entire computer. Each wave or cycle is called a Hertz (Hz).
The entire computer has a beating heart not of blood but of electricity. We measure the number of cycles as Gigahertz (GHz), Megahertz (MHz), Kilohertz (kHz), etc.
When this electricity is beating we can sense a low voltage or a high voltage. This is 0 or 1. High voltage is 1 and low voltage is 0. We can even set the 0s and 1s into the hardware and cause it to memorize data.
But it is impossible to use that memorized data without turning on the beating electricity. The flow of this electricity is an algorithm. In the end we are looking at a living, breathing physical algorithm. A machine.
It can’t run without its own electricity and because of that algorithms and memory are inseparable. You must use an algorithm to make the computer function. So you will never go without both.
Abstract data structures
There are too many data structures out there. To memorize them would make you a savant. But it is not necessary.
Abstract data structures(Abstract Data Types) are the concept behind the implementation. The abstraction of MegaInt before would be the Abstract Data Type “wrapper”.
Wrappers add functionalities to primitives as a new class. There are others out there like:
- List
- Map
- Set
- Tensor
- Graph
- Etc.
Every programming language has several implementations of every Abstract Data Type out there.
Common algorithms
If you go to college you will be taught common algorithms. It will be a difficult semester as you write pseudo code and memorize solutions.
However, the best way to learn algorithms is by self-discovery and reading code. We discover the solution while writing. We learn others by reading.
Most advanced developers do not memorize the pseudo code of algorithms. They find it themselves and memorize it out of pride of discovery. Here are some common algorithms:
- Binary Search
- Merge Sort
- Depth First Search
- Dynamic Programming
- Many more…
These are the typical algorithms we use in competitive programming and algorithms class. But for me I noticed I used none of those. Always I find a simpler solution to be the best, even performing better than runtime.
Once a few years ago I implemented binary search for a backend. The array was always above size 50. I thought it was the bomb at 12 lines of code. My code reviewer looked at it and said I could write it brute force with 2 lines.
After reducing the function to two lines the brute force was 1/4 faster in all cases. Wow, binary search didn’t even matter. So far in real world programming I used none of these algorithms.
How do you learn data structures and algorithms?
Instead of learning algorithms what I like to learn is techniques. Techniques are what make you a better problem solver. As an example some techniques-like in graphics programming are these:
- Rasterization
- Shading
- Texture mapping
- Level of Detail Rendering
- Etc.
All niches have techniques. These are the true algorithms which we use day to day. Lets take two more for web development and machine learning. Here is web development:
- Routing
- JWT authorization
- Responsive web design
- DOM manipulation
And machine learning:
- Supervised learning
- Unsupervised learning
- Regression
- Transfer Learning
- Etc.
We focus on these and get better results than learning general algorithms ever could. Techiques are what is used in the real world.
How to learn data structures
There are infinitely less Abstract Data Types than there are implementations. As programmers it is our job to memorize the ADT(Abstract Data Type).
When we associate the class to an ADT in our head we readily understand it without looking at the code. We can learn all implementations based on the ADT concept itself.
Lets say you have this code:
public class MyList<T> {
private Object[] elements;
private int size;
private static final int DEFAULT_CAPACITY = 10;
public MyList() {
elements = new Object[DEFAULT_CAPACITY];
size = 0;
}
public void add(T element) {
if (size == elements.length) {
ensureCapacity();
}
elements[size++] = element;
}
public T get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of bounds: " + index);
}
return (T) elements[index];
}
public int size() {
return size;
}
private void ensureCapacity() {
int newCapacity = elements.length * 2;
elements = Arrays.copyOf(elements, newCapacity);
}
}
This is the “List” Abstract Data type in Java. It wraps around an array and makes it able to change size.
There are two ways to tackle learning something like this. Either we read the code and learn what each function does. Or we refer to the functions of the List ADT.
The List class has been written hundreds of times in all languages. Should we memorize 100 instances of List or 1 abstraction of List? No we learn the abstraction.
That is how we learn data structures. The abstractions are what is taught in the Data Structures & Algorithms college courses.
Resources to learn data structures and algorithms
There are several types of material for learning data structures & algorithms. Books, online courses, college, language models, frameworks and LeetCode are the main ones.
Language models
For learning DSA (Data Structures & Algorithms) we should always use a language model. No matter which way we are learning DSA we use language models.
Other resources
I tried learning DSA from Udemy. It was a failure. I kept zoning out and not knowing what to code after watching the lecture. It seems writing code is the best way to learn these things.
I am not a fan of learning DSA from books or online courses. Instead what I am a fan of is using frameworks, coding and LeetCode. The majority of my DSA learning came from writing code and LeetCode solving. I used resources like NeetCode, the Grind129 and just plain using Java to learn everything.
College was helpful but more to keep myself passionate. In the end, like most things programming-implementation is king.